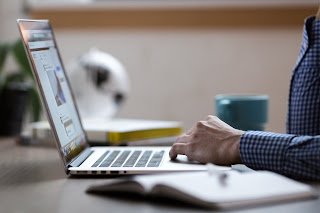
How to dockerize a Spring Boot application
Dockerizing a Spring Boot application is an essential skill for software developers, as it enables the efficient deployment and management of applications in a containerized environment.
Embark on the journey of Dockerizing your Spring Boot application—a must-have skill for any software developer seeking streamlined deployment and effortless management in a containerized realm.
By encapsulating your Spring Boot masterpiece into a Docker container, you unlock a world of benefits: seamless behavior across diverse platforms, effortless scalability, and turbocharged development.
Let this guide be your trusted companion as you navigate through the process of Dockerization. From crafting the perfect Dockerfile to fine-tuning essential configurations, to finally unleashing your container into the digital wild—every step promises to elevate your Spring Boot application's performance and upkeep.
To execute these actions, you’ll need:
No comments:
Post a Comment